Capacitance Meter
In order to test an electronic circuit, we need some testing equipment. Capacitance meter is an electronic equipment used to measure capacitance. This project will show how to construct a simple capacitance meter using 8051 controller.
Theory & Design
Capacitance can be measured using the RC circuit. In this project, we have designed the capacitance meter using RC circuit,comparator and microcontroller.
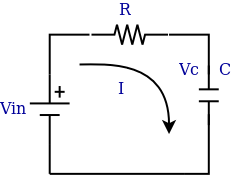
Voltage across the capacitor(`V_c`) at any instant of time during the charging period is given as,
`V_c = V_i (1-e^(-t/(RC)))`
where,
`V_c` - Voltage across the capacitor
`V_i` - Supply voltage
`t` - is the elapsed time since the application of supply voltage
`RC` - is the time constant of RC charging circuit
The time required to charge the capacitor to 63.5 percent of input voltage is known as time constant (`tau`), as given by
`tau = RC`
The capacitance is given by the following formula:
`C = tau / R`
What You Need
-
ZKit-51 Motherboad
-
Comparator IC LM339N - 1no.
-
Transistor 2N3906 - 1no.
-
Resistor 51k - 1no.
-
Resistor 10k - 1no.
-
Resistor 1k - 1no.
-
Resistor 470 - 1no.
-
Variable resistor 100k - 2nos.
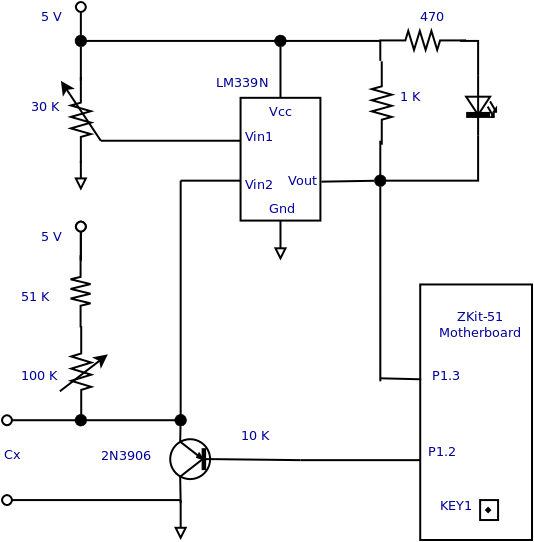
Working Principle
Transistor is used to discharge the capacitor before starting the measurement.Variable resistor near the comparator is used to set the voltage to 63.2 percent of input voltage.
-
Initially the base of the transistor should be made low to discharge the capacitor.
-
After the pressing the key1, base of the transister will be made high.
-
So that the capacitor starts charging.
-
Charging time of the capacitor is calculated using stop watch APIs.
-
When the voltage across the capacitor reaches 63.2 percent of input voltage, comparator triggers a signal.
-
After receiving the signal from the comparator, stop watch will be stopped.
-
Using the above formula, capacitance is calculated.
Software
The code for measuring capacitance and displaying it on LCD, using ZDev library is given below.
-
/static/code/zkit-51-cap-meter.c[Download the source file]
-
/static/code/zkit-51-cap-meter.ihx[Download the hex file for ZKit-51!]
-
/static/code/swatch.c[Downlod the source file for stopwatch]
/**
*
* code for measuring the capacitance value and to display
* it on lcd using zkit-51 motherboard and zdev library
*
**/
#include<stdio.h>
#include <board.h>
#include <lcd.h>
#include <swatch.h>
#define KEY1 P2_0
/* resistor value across unknown capasitor is 151K */
#define TOTAL_RESISTANCE 156
#define LM3339_PIN_CAPCITANCE 63
void putchar(char val)
{
lcd_putc(val);
}
uint8_t check_key()
{
if(!KEY1)
return 1;
else
return 0;
}
int main()
{
uint32_t time_us;
uint32_t cap_value;
lcd_init();
swatch_init();
board_init();
P1_2=0;
while(check_key()==0);
P1_2=1;
swatch_start();
while (P1_3 == 0);
swatch_stop();
time_us=swatch_get_time();
if (time_us>TOTAL_RESISTANCE) {
cap_value=(time_us)/TOTAL_RESISTANCE;
printf("%ld%s",cap_value," nf");
} else {
cap_value=(time_us*1000)/TOTAL_RESISTANCE;
printf("%ld%s",cap_value - LM3339_PIN_CAPCITANCE," pf");
}
return 0;
}