Stepper Motor Board with ZIO
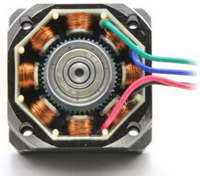
In this, third installment in the stepper motor seris, we are going to learn about interfacing a stepper motor to the ZIO board. If you haven’t, you might want to read the stepper motor fundamentals described in the blog article Stepper Motor, Getting Started, before reading this article.
Hardware components
-
Stepper Motor Board
-
ZIO Mother Board
-
Stepper Motor
-
12V Power Supply
Hardware Setup
-
Connect the Stepper Motor Board to the ZIO mother board’s DIO header.
-
Connect the Stepper Motor to the Stepper Motor Board.
-
Connect the 12V supply to the Stepper Motor Board.
Software
"""Stepper motor interface with ZIO."""
import zio
import time
agent = zio.Agent("/dev/ttyACM0")
gpio = zio.GPIO(agent)
ON = 1
OFF = 0
# Pin map to stepper motor signal.
A1 = 12
A2 = 13
B1 = 14
B2 = 15
# Wave drive mode.
pins = [A1, B1, A2, B2]
while True:
for phase in pins:
gpio.write_pin(phase, ON)
time.sleep(0.5)
gpio.write_pin(phase, OFF)
ZIO GPIO pins mapping for each of the stepper motor signals is shown in the following diagram.
Pin No. | DIO pins | Phase |
---|---|---|
0 |
12 |
|
1 |
13 |
|
2 |
14 |
|
3 |
15 |
|
The pin mapping is stored in an array pins
, so that it can be easily
iterated over.
In the infinite while
loop, the stepper motor is driven in wave
drive mode, with one phase ON
. The sequence table is given
below. The phases indicated with a *, are driven 12V, while the other
phases are connected to ground.
Pin No. | Phase | Step 1 | Step 2 | Step 3 | Step 4 |
---|---|---|---|---|---|
0 |
|
* |
|||
1 |
|
* |
|||
2 |
|
* |
|||
3 |
|
* |
Credits
-
The stepper motor icon is based on the original image located here, at Wikimedia Commons.