Currency converter using J2ME
The article Getting Started with J2ME showed how to build a simple Hello World application using J2ME. This article takes up from where the previous article left off and shows how to build a complete application, a currency converter, using the J2ME framework. The currency converter is a simple, yet complete application that converts from Indian Rupees (INR) to US Dollars (USD).
Background
In this article, we show how to create the user interface (GUI)
elements of a MIDlet. MIDP 2.0 provides UI classes in the package
javax.microedition.lcdui
. This package has a class named Display
.
The Display
class provides methods to retreive information about the
display and to show/change the current GUI element that you want
display. A MIDlet shows a Displayable
UI element on a Display using
the setCurrent(Displayable element)
method of the Display class.
Alerts
Alerts are used to display information or error messages that stay on the screen for a short period of time and disappears. You can control several aspects of an alert by calling the relevant methods or using the right constructor.
Commands
A MIDlet interacts with a user through commands. A command is the
equivalent of a button in a normal application, and can only be
associated with a displayable GUI element. The Displayable
class
allows the user to attach a command to it by using the method
addCommand(Command command)
. Displayable GUI element can have
multiple commands associated with it.
Command Listener
The responsibility for acting on commands is performed by a class
implementing the CommandListener
interface, which has a single
method commandAction(Command com, Displayable dis)
. However before
command information can travel to a listener, the listener is
registered with the method setCommandListener(CommandListener
listener)
from the Displayable
class like Form
, List
and etc.
TextField
The TextField
class is used to capture one line or multiple lines of
text entered by the user. The number of lines of a text field depends
on the maximum size of the text field when you create an instance of
the TextField
class.
Form
A Form
is a collections of instances of the GUI elements like
TextField
, StringItem `and etc. `Form
is a sub-class of
Displayable
.
GUI Elements can be added to the form by using the append(Item item)
method, commands can be added using the addCommand(Command command)
method. We can create more than one form in a application but only one
form is displayed at a time, we also can set command Listener to a
Form
by using setCommandListener(CommandListener listener)
.
Source code
import javax.microedition.lcdui.*;
import javax.microedition.midlet.*;
public class CurrencyMidlet extends MIDlet implements CommandListener {
private Display display;
private Form Input;
private TextField inr;
private Command convert;
public CurrencyMidlet() {
display = Display.getDisplay(this);
Input = new Form("INR to USD Converter");
inr = new TextField("Enter INR:", null, 10, TextField.DECIMAL);
convert = new Command("Convert", Command.SCREEN, 1);
Input.append(inr);
Input.addCommand(convert);
Input.setCommandListener(this);
}
public void startApp() {
/* Display form */
display.setCurrent(Input);
}
public void pauseApp() {
/* pass */
}
public void destroyApp(boolean unconditional) {
/* pass */
}
public void commandAction(Command c, Displayable d) {
String text = null;
long rs = 0;
int paise = 0;
long dollor = 0;
long cent = 0;
String final_dollar = null;
if (c == convert){
text = inr.getString();
paise = text.indexOf('.');
if (paise == -1) {
rs = Long.parseLong(text) * 100;
} else {
rs = Long.parseLong(text.substring(0, paise)) * 100;
paise = Integer.parseInt(text.substring((paise+1), text.length()));
rs = rs + paise;
}
dollor = rs * 100 / 4470;
cent = dollor % 100;
dollor = dollor / 100;
final_dollar = String.valueOf(dollor) + "." + String.valueOf(cent);
Alert alert = new Alert("US Dollor", final_dollar + "$", null, AlertType.INFO);
alert.setTimeout(Alert.FOREVER);
display.setCurrent(alert);
}
}
}
In the above Java file a Form object Input
is created. In our
application one TextFiled inr
(Getting INR as input from the user )
and one Command convert
are added to the Form Input. Then
Input.setCommandListener(this)
method will expect a command input
from the command button.
The public CurrencyMidlet()
is the constructor of the class
CurrencyMidlet. All the initialisation is performed inside the
constructor.
After the initialisation the startApp()
method is invoked. This
method displays the Input
form by calling
display.setCurrent(Input)
.
Now we should enter the INR value in the TextField
inr
and the
press convert button. When we press convert button the command
listener automatically calls the method commandAction(Command c,
Displayable d)
.
The action to be taken after pressing the command button is defined
inside the commandAction()
method. When command button is pressed
the corresponding USD value is displayed on the screen.
J2ME doesn’t support Floating point operation. So Fixed-point arithmetic is used.
Running the App
-
Shown below is the screenshot of the app. running in the emulator.
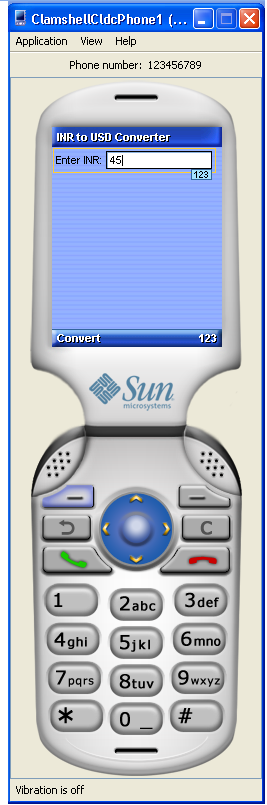
-
Install /static/code/Test.jar[CurrencyMidlet.jar] and /static/code/Test.jad[CurrencyMidlet.jad] in your mobile and run the app.